There are a lot of features newly introduced in Selenium 4. We can capture screenshots of the entire page in Selenium 3, but with the help of Selenium 4, we can capture screenshots of a specific web element. The screenshot function in Selenium WebDriver 4 allows us to take screenshots at the element, section, and full-page levels. The following sections briefly describe the different methods for screenshot capture in Selenium 4.
- Taking a screenshot of an element
There is a new method named getScreenshotAs() in Selenium 4. With this method, we can capture a screenshot of a specific web element.
To take a screenshot of an element, we need to first find the element. The below code finds the element by its class name. Then take a screenshot using the reference to that particular element. In this case, you get a screenshot of only the element we have chosen.
For example, Below is the code snippet to find the screenshot of the banner video of the website https://www.terrificminds.com/.
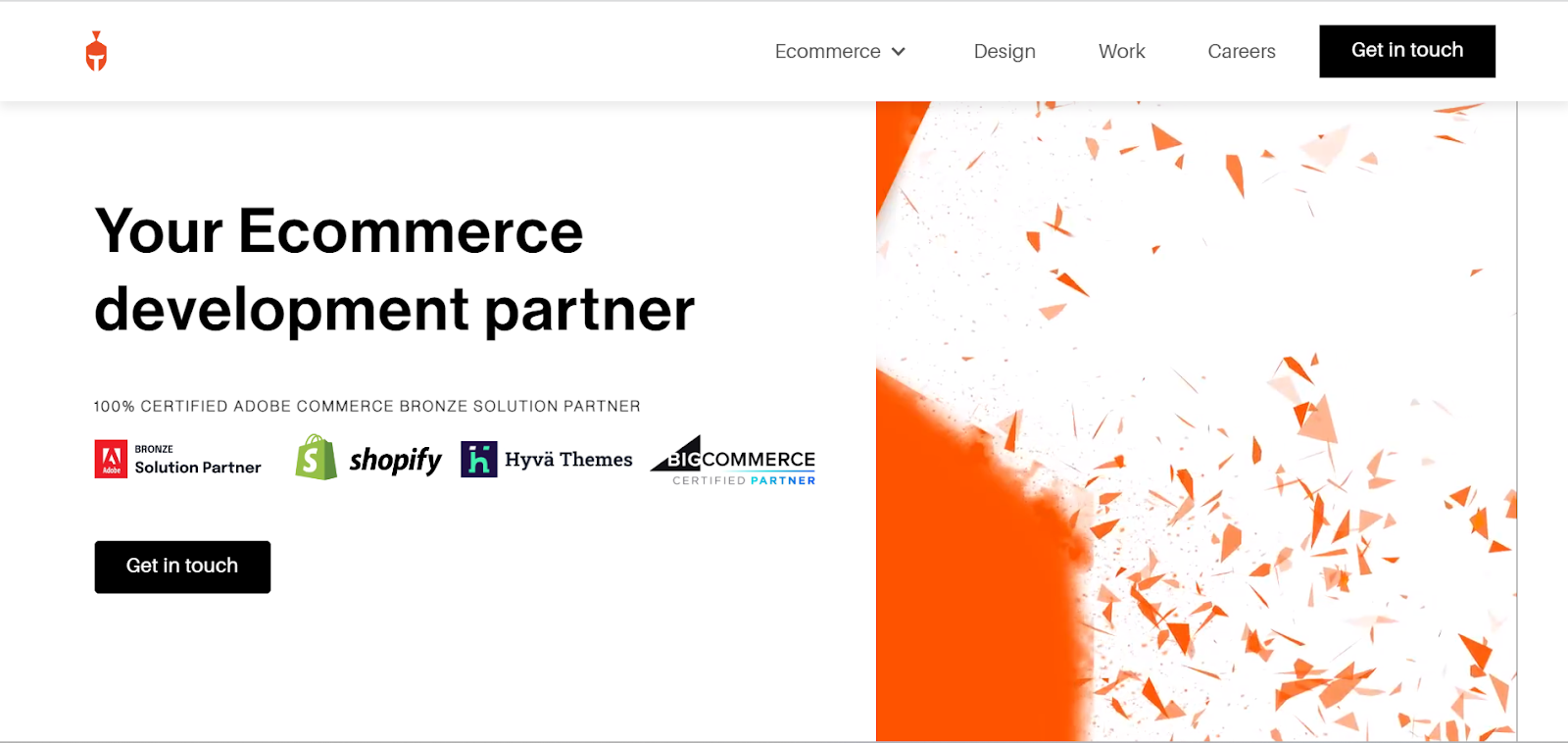
<Code snippet for element-level screenshot>
public void takeElementScreenshot() {
WebElement elementBanner = driver.findElement(By.className("elementor-video"));
File source = elementBanner.getScreenshotAs(OutputType.FILE);
File dest =new File("./screenshots/elementvideo.png");
FileHandler.copy(source, dest);
}}
- Taking screenshots of a section
We can take a screenshot of a section, as shown below in the code snippet. If we want to check the header section, the code is as follows.

public void takeSectionScreenshot() throws InterruptedException {
WebElement elementSection= driver.findElement(By.xpath(“//div[@data-elementor-type='header']");
File source = elementSection.getScreenshotAs(OutputType.FILE);
File dest =new File("./screenshots/header.png");
FileHandler.copy(source, dest);
}}
- Taking a screenshot of a full page
For Firefox, a method called getFullPageScreenshotAs() exists in Selenium 4. It enables us to capture a screenshot of an entire page and save it to the designated location.
Rather than typecasting it to the 'TakeScreenshot' interface in this instance. For the instance of "FirefoxDriver," we must typecast it. Only the Firefox browser supports it.
Following code allows you to take a full-page screenshot of the https://www.terrificminds.com/ website from top to bottom.
public void captureFullPageScreenshot() throws IOException {
File src = ((FirefoxDriver)driver).getFullPageScreenshotAs(OutputType.FILE);
FileHandler.copy(src, new File("./screenshots/FullPageScreenshot.png"));
}
-
Taking screenshots of only visible part of the page
The following code allows the user to take a screenshot of the page that is currently visible. This method exists in Selenium 3.
File src = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE);
FileHandler.copy(src, new File("./screenshots/PageScreenshot.png"));
}}
Conclusion
This blog explains how to use Selenium 4's new screenshot functionality to capture screenshots at various UI levels. This feature can be used by testers for bug reporting and debugging, and it is really useful for automation testers. In case of a test failure, we can send an email notification to the team, including the screenshot facility.
To read about the method of sending email notifications for failed test cases with screenshots attached in Selenium 3 and Cypress, please refer to this blog.